1. 自定义组件间也可以和普通标签(<div> <span>)一样指定属性传参
2. 形如(<child value="hello coolight"></child>)给组件<child>指定value属性为"hello coolight"
3. 我们也可以自己模拟v-model的效果
下面我们来聊聊怎么传参、接收与更新
目录
传参,v-bind
- 子组件接收参数需要使用一个函数defineProps()
- defineProps():
- 不需要import导入即可使用
- 只能在<script setup>中使用
- 不可以在局部变量中使用这个函数
- 不可以访问 <script setup> 中定义的其他变量
- 需要传入自定义参数名,[可选]指定参数类型,[可选]指定参数是否必须传入,[可选]指定参数的默认值。
- 类型:
- Number
- Boolean
- String
- Function
- Object
- Array
- ...
- 类型:
- 返回组件的接收所有参数构成的一个对象
- 当类型不对或是指定必须传入而没有传入时,vue将会有警告,报错
- 必须传入但没有传入:

- -
- 传入类型错误:
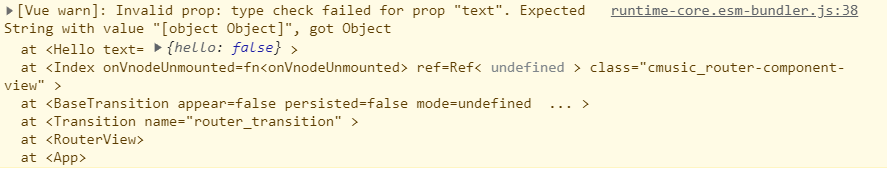
- 示例:
- 组件:
<script setup>
import { toRefs } from 'vue';
const props = defineProps({
text:{
type:String,
default:"默认:hello coolight",
required:false
}
})
const {
text,
} = toRefs(props);
</script>
<template>
<span>{{props.text}} - {{text}}</span>
</template>
- -
- 使用组件
<script setup>
import helloVue from '../../components/global/hello.vue';
</script>
<template>
<div>
<hello-vue />
<hello-vue text="洛天依"/>
<hello-vue :text="'v-bind:洛天依'" />
</div>
</template>
- 解析:
- 组件定义了一个要接收的参数text,并指定了它的类型为字符串String,默认值,并且它并不是必须传入的。
- 组件的<template>中,可以通过props访问参数props.text,也可以使用toRefs,解构出来。
- toRefs()需要import
- 如果不使用toRefs(),直接解构将导致参数失去响应性
- 在使用组件时,可以直接指定参数并传参,也可以使用v-bind,动态传参。
- 运行结果:

v-model
一般的传参进来,组件对它是只读的,不能修改。
只读也并不完全,对于数组和对象是可以操作其内部值的。
但我们可以使用v-model指定参数,使之允许组件来修改它。
”只读“的探讨
- 这个只读限制和const声明一样。
- 只读是针对变量本身的指向,如果你了解c/c++的指针则会很好理解,它限制了变量的指向,但对于变量指向的内容是可以操作的。
- 比如对数组、对象的内部变量的操作则是可以的,但不能让变量指向别的数组或对象,如 list = [],就是直接让list变量重新指向新的空数组[]。
- 示例:
- 组件:
<script setup>
import { reactive } from 'vue';
import { toRefs } from 'vue';
const props = defineProps({
obj:{
type:Object
}
})
const {
obj,
} = toRefs(props);
const btn1_click = () => {
props.obj.hello = "coolight - 1";
}
const btn2_click = () => {
props.obj = reactive({
hello:"coolight - 2"
})
}
</script>
<template>
<div>
<button @click="btn1_click">1: {{obj.hello}}</button>
<button @click="btn2_click">2: {{obj.hello}}</button>
</div>
</template>
- -
- 使用组件:
<script setup>
import { reactive } from 'vue';
import helloVue from '../../../components/global/hello.vue';
let obj = reactive({
hello:"洛天依"
})
</script>
<template>
<div>
<span>span: {{obj.hello}}</span>
<hello-vue :obj="obj" />
</div>
</template>
- 注意:
- 在使用组件的代码中,obj被使用reactive()进行赋值,因此获得了响应性,如果直接定义为一个对象,obj就没有响应性,后续无论在父子组件中修改obj,html页面的显示内容都不会改变。
- 运行结果:

- 点击btn2时修改失败,触发警告,提示变量obj是只读的:

使用v-model
- 使用v-model则可以解决上述问题
- 在这之前,组件内需要定义一个更新信号,“update:valueName”,其中的valueName为参数名
- 我们上面的例子里的参数是obj,因此信号就是 “update:obj”
- 使用defineEmits()可以定义信号,而它也是我们后面自定义事件的重点。
- 发出修改信号 emits("信号名", 传参);
- 示例:
- 组件:
<script setup>
import { toRefs } from 'vue';
const props = defineProps({
obj:{
type:Object
}
})
const emits = defineEmits ({
'update:obj':null //null位置可以是一个检查函数,用以检查绑定这个信号的处理函数是否有接收参数等,这里就不需要了
});
const {
obj,
} = toRefs(props);
const btn1_click = () => {
props.obj.hello = "btn1";
}
const btn2_click = () => {
emits('update:obj', {
hello:"btn2"
});
}
</script>
<template>
<div>
<button @click="btn1_click">{{obj.hello}} - 修改为 btn1</button>
<button @click="btn2_click">{{obj.hello}} - 修改为 btn2</button>
</div>
</template>
- -
- 使用组件:
<script setup>
import { reactive } from 'vue';
import helloVue from '../../../components/global/hello.vue';
let mValue = reactive({
obj:{
hello:"洛天依"
},
})
</script>
<template>
<div>
<span>span: {{mValue.obj.hello}}</span>
<hello-vue v-model:obj="mValue.obj"/>
</div>
</template>
- 注意:
- 即使使用了v-model,我们也不能直接在组件中用=修改obj(如obj = { hello:"wow" } 是不行的)
- 需要修改obj时,使用emits()发出信号即可
- 注意在使用组件的代码中,我们使用mValue包裹了obj后再传递给了子组件,因为如果同上一个例子中,直接声明obj后传递,在修改时会发生一些问题。因此建议把它放reactive()内。
- 运行结果:
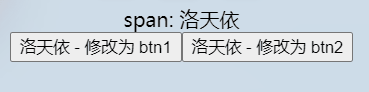
尝试取代v-model
- 在上面的例子里,我们已经知道,修改obj时是发出了一个信号"update:obj"
- 那么我们就可以自己绑定一个事件给这个信号,然后自己来更新obj
- 示例(组件代码不变,使用组件的代码修改):
<script setup>
import { reactive } from 'vue';
import helloVue from '../../../components/global/hello.vue';
let mValue = reactive({
obj:{
hello:"洛天依"
},
})
const change = (in_obj) => {
console.log("我们的change");
mValue.obj = in_obj;
}
</script>
<template>
<div>
<span>span: {{mValue.obj.hello}}</span>
<!-- 也可以这样:
<hello-vue v-model:obj="mValue.obj" @update:obj="change"/>
-->
<hello-vue :obj="mValue.obj" @update:obj="change"/>
</div>
</template>
- 当我们自己实现更新事件时,使用v-model和v-bind绑定是一样的
- 运行后,无论使用了v-model还是v-bind,都会执行我们的更新事件change()
- 有时候我们传进去的值是getter生成的等各种原因,直接v-model绑定在修改时会出错,因此我们就需要自己实现变量的更新事件。
Hi, I do think this is a great blog. I stumbledupon it 😉 I am going to return yet again since i have bookmarked it. Money and freedom is the best way to change, may you be rich and continue to help other people.
price of prednisone tablets prednisone ray pharm prednisone 200 mg tablets
Узнай все о признаки варикоцеле рецидив варикоцеле
buy priligy: dapoxetine online – max pharm
Right here is the perfect blog for anybody who wants to find out about this topic. You understand a whole lot its almost hard to argue with you (not that I personally will need to…HaHa). You definitely put a new spin on a topic which has been written about for many years. Excellent stuff, just excellent.
Узнай все о варикоцеле 1 степени варикоцеле
how can i get generic clomid price rex pharm buy cheap clomid price
Узнай все о варикоцеле слева варикоцеле у мужчин
prednisone online pharmacy: ray pharm – prednisone 10mg tablets
Узнай все о признаки варикоцеле варикоцеле причины
items4games
Discover a Realm of Interactive Possibilities with Items4Games
At Items4Play, we deliver a dynamic platform for enthusiasts to acquire or exchange gaming accounts, virtual items, and features for top titles. If you’re seeking to upgrade your gaming arsenal or wanting to profit from your profile, our service provides a smooth, safe, and profitable process.
Why Select ItemsforGames?
**Extensive Title Catalog**: Browse a vast array of titles, from thrilling adventures such as Warzone and Call of Duty to captivating role-playing games such as ARK and Genshin. We have all games, making sure no gamer is excluded.
**Range of Features**: Our products include account purchases, in-game currency, exclusive goods, achievements, and mentoring sessions. If you want assistance gaining levels or getting exclusive benefits, we’ve got you covered.
**Easy Navigation**: Browse effortlessly through our structured marketplace, arranged by order to locate precisely the item you need with ease.
**Safe Deals**: We ensure your safety. All trades on our platform are conducted with the utmost security to secure your private and payment details.
**Key Points from Our Collection**
– **Action and Survival**: Games like ARK: Survival Evolved and Survival Day give you the chance to explore tough environments with top-notch items and passes on offer.
– **Adventure and Adventure**: Elevate your gameplay in games like Clash Royale and Wonders Age with in-game currencies and services.
– **eSports Play**: For competitive players, enhance your abilities with training and level-ups for Valorant, Dota 2, and LoL.
**A Hub Designed for Players**
Supported by ApexTech, a reliable business officially recognized in Kazakh Nation, Items4Games is a market where video game wishes are realized. From buying advance keys for the newest games to finding rare virtual goods, our platform caters to every gaming need with skill and efficiency.
Join the group right away and elevate your game play!
For support or help, reach out to us at **support@items4games.com**. Let’s all improve the game, as one!
dapoxetine online: priligy maxpharm – buy priligy
Узнай все о варикоцеле причины варикоцеле симптомы
Предлагаем услуги профессиональных инженеров офицальной мастерской.
Еслли вы искали официальный сервисный центр xiaomi, можете посмотреть на сайте: официальный сервисный центр xiaomi
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Узнай все о варикоцеле у мужчин симптомы варикоцеле симптомы
Узнай все о варикоцеле слева варикоцеле яичка
king buffalo
king buffalo скачать
cost generic clomid without a prescription: buy clomid – can i purchase clomid without prescription
how to buy amoxicillin online http://priligymaxpharm.com/# buy dapoxetine online
generic amoxicillin online https://amoxilcompharm.com/# amoxicillin online canada
An impressive share! I’ve just forwarded this onto a colleague who had been doing a little research on this. And he in fact ordered me breakfast because I stumbled upon it for him… lol. So allow me to reword this…. Thank YOU for the meal!! But yeah, thanx for spending the time to discuss this subject here on your website.
buying amoxicillin in mexico: Com Pharm – amoxicillin 500 mg brand name
amoxicillin over the counter in canada amoxil com pharm amoxicillin online purchase
Explore a Universe of Interactive Chances with ItemsforGames
At ItemsforGames, we deliver a vibrant marketplace for players to purchase or exchange accounts, goods, and services for widely played video games. Whether you are seeking to enhance your gaming arsenal or interested in selling your game account, our platform offers a smooth, secure, and rewarding experience.
Why Choose Items4Games?
**Extensive Title Library**: Explore a large array of video games, from action-packed adventures like Battleground and COD to engaging RPGs like ARK and Genshin Impact. We include all games, guaranteeing no gamer is left behind.
**Variety of Features**: Our offerings cover account purchases, credits, rare collectibles, achievements, and coaching options. Whether you want assistance leveling up or unlocking special rewards, we have it all.
**Easy Navigation**: Browse easily through our well-organized platform, organized in order to get exactly the game you need quickly.
**Protected Exchanges**: We ensure your protection. All trades on our site are processed with the top protection to secure your confidential and financial data.
**Highlights from Our Collection**
– **Survival and Exploration**: Titles ARK and Day-Z give you the chance to dive into tough worlds with top-notch items and passes available.
– **Adventure and Questing**: Boost your performance in games such as Clash Royale and Wonders Age with virtual money and options.
– **eSports Gaming**: For serious players, enhance your skills with coaching and account upgrades for Val, Dota 2, and LoL.
**A Marketplace Made for Gamers**
Backed by Apex Technologies, a trusted business registered in Kazakh Nation, Items4Play is a place where playtime dreams come true. From acquiring pre-order passes for the freshest releases to locating unique virtual goods, our marketplace meets every gamer’s wish with skill and speed.
Join the group today and upgrade your gaming play!
For support or assistance, email us at **support@items4games.com**. Let’s game better, as one!
Предлагаем услуги профессиональных инженеров офицальной мастерской.
Еслли вы искали официальный сервисный центр xiaomi, можете посмотреть на сайте: сервисный центр xiaomi в москве
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
I must thank you for the efforts you have put in writing this blog. I’m hoping to view the same high-grade content from you in the future as well. In fact, your creative writing abilities has motivated me to get my own, personal website now 😉
Предлагаем услуги профессиональных инженеров офицальной мастерской.
Еслли вы искали сервисный центр xiaomi, можете посмотреть на сайте: сервисный центр xiaomi в москве
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
prednisone tablets canada: raypharm – prednisone without a prescription
how to get generic clomid pills buy generic clomid without prescription how can i get generic clomid for sale
Узнай все о двустороннее варикоцеле клиника варикоцеле
non prescription prednisone 20mg: Prednisone Without Prescription – otc prednisone cream
This is fantastic! Full of useful information and highly well-written. Thanks for offering this.
слот king buffalo
игровой аппарат king buffalo
Узнай все о варикоцеле причины варикоцеле диагностика
king buffalo
king buffalo скачать
amoxicillin 500mg buy online canada http://clomidrexpharm.com/# where can i get generic clomid now
Узнай все о варикоцеле причины возникновения варикоцеле и потенция
Узнай все о варикоцеле яичка варикоцеле 3 степени
amoxicillin 250 mg capsule http://prednisoneraypharm.com/# india buy prednisone online
Узнай все о варикоцеле яичка варикоцеле симптомы
prednisone without rx: Prednisone Without Prescription – prednisone prescription for sale
Узнай все о рецидив варикоцеле варикоцеле 3 степени
Узнай все о варикоцеле симптомы степени варикоцеле
Узнай все о признаки варикоцеле степени варикоцеле
buy priligy: priligy maxpharm – buy priligy
Judul: Merasakan Pengalaman Bermain dengan “PG Slot” di Situs Kasino ImgToon.com
Dalam alam permainan kasino online, mesin slot telah menjadi salah satu permainan yang paling diminati, terutama jenis PG Slot. Di antara beberapa situs kasino online, ImgToon.com adalah tujuan utama bagi peserta yang ingin menguji peruntungan mereka di banyak permainan slot, termasuk beberapa kategori populer seperti demo pg slot, pg slot gacor, dan RTP slot.
Demo PG Slot: Menjalani Tanpa Risiko
Salah satu fitur menarik yang diberikan oleh ImgToon.com adalah demo pg slot. Fitur ini mengizinkan pemain untuk memainkan berbagai jenis slot dari PG tanpa harus memasang taruhan nyata. Dalam mode demo ini, Anda dapat menguji berbagai taktik dan memahami proses permainan tanpa ancaman kehilangan uang. Ini adalah cara terbaik bagi pemain baru untuk terbiasa dengan permainan slot sebelum beralih ke mode taruhan nyata.
Mode demo ini juga memberi Anda gambaran tentang potensi kemenangan dan hadiah yang mungkin bisa Anda dapatkan saat bermain dengan uang nyata. Pemain dapat menjelajahi permainan tanpa cemas, membuat pengalaman bermain di PG Slot semakin membahagiakan dan bebas beban.
PG Slot Gacor: Kesempatan Besar Mencapai Kemenangan
PG Slot Gacor adalah sebutan terkemuka di kalangan pemain slot yang mengacu pada slot yang sedang dalam fase memberi kemenangan tinggi atau lebih sering diistilahkan “gacor”. Di ImgToon.com, Anda dapat menemukan berbagai slot yang ada dalam kategori gacor ini. Slot ini dikenal memiliki peluang kemenangan lebih tinggi dan sering memberikan bonus besar, membuatnya pilihan utama bagi para pemain yang ingin memperoleh keuntungan maksimal.
Namun, perlu diingat bahwa “gacor” atau tidaknya sebuah slot dapat bergeser, karena permainan slot tergantung generator nomor acak (RNG). Dengan melakukan permainan secara rutin di ImgToon.com, Anda bisa mengidentifikasi pola atau waktu yang tepat untuk memainkan PG Slot Gacor dan menambah peluang Anda untuk menang.
RTP Slot: Faktor Penting dalam Pencarian Slot
Ketika mendiskusikan tentang slot, istilah RTP (Return to Player) adalah faktor yang sangat krusial untuk dihitung. RTP Slot berkaitan pada persentase dari total taruhan yang akan dipulangkan kepada pemain dalam jangka panjang. Di ImgToon.com, setiap permainan PG Slot disertai dengan informasi RTP yang terperinci. Semakin tinggi persentase RTP, semakin besar peluang pemain untuk mendulang kembali sebagian besar dari taruhan mereka.
Dengan memilih PG Slot yang memiliki RTP tinggi, pemain dapat memaksimalkan pengeluaran mereka dan memiliki peluang yang lebih baik untuk menang dalam jangka panjang. Ini menyebabkan RTP sebagai indikator krusial bagi pemain yang mencari keuntungan dalam permainan kasino online.
buy priligy: cheap priligy – dapoxetine online
buy dapoxetine online max pharm priligy
buy generic clomid: clomid online – cost of generic clomid without dr prescription
Тут можно преобрести купить огнеупорный сейф несгораемый сейф цена